Enterprise Integration
This use case could be seen as the super set of all previous use cases but that would be too simple. Once JunC++ion is part of your toolkit and you have purchased an enterprise-wide deployment license, you have so many fewer constraints when it comes to choosing products that you can start reaping benefits beyond the specific API integration.
You might already have gotten an idea about the benefits of language-neutral development via .NET, but that has always been delivered by locking you into Microsoft's implementations.
What if you could largely ignore the language boundaries between Java and C++? What if you could combine Java's platform-neutrality with JunC++ion-enabled language-neutrality?
So your enterprise bus is written in Java and you have many C++ applications. Not a problem.
Why not use your standard JMS provider for connecting your C++ client application to a server?
The other group wrote a validation library in Java. Couldn't we use that in our C++ app?
We have this high performance C++ library. Can we create a reliable Java wrapper for it?
Being able to leverage free Java products in your C++ applications can allow you to reduce the number of third-party products you rely on. That can translate into tremendous cost savings over time that go far beyond the mere tool benefit!
And before we move on to some sample code, allow us a final quick pitch for Codemesh's JuggerNET product: how about adding .NET to the mix?
What a JDBC Integration Might Look Like
Why would oyu want to use a JDBC driver from a C++ application? Well, all database drivers have bugs, they have feature set limitations, they support different point releases, they might require certain C++ build options, etc.
When you already have Java applications that successfully use a database via particular a JDBC driver, being able to use the same driver in your C++ applications can represent a significant reduction in cost and complexity, as well as improved portability.
This entire premise hinges on the availability of high-quality C++ bindings for the JDBC API. JunC++ion can create those for you, allowing you to write code like this:
Connection conn = null;
// we execute our entire Java code in a try block and catch any exceptions
// that might be thrown at this level. In a more sophisticated application
// you might have local try/catch clauses in other places.
try {
std::string db = std::string( "jdbc:hsqldb:file:" ) + get_example_dir() + "/sampledb";
String user = "SA";
String password = "";
conn = DriverManager::getConnection( db.c_str(), user, password );
std::cerr << "Connected to DB!" << std::endl;
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery( "SELECT FIRSTNAME, LASTNAME FROM CUSTOMER" );
while( rs.next() ) {
String firstName = rs.getString( "FIRSTNAME" );
String lastName = rs.getString( "LASTNAME" );
std::cout << firstName.to_chars() << " " << lastName.to_chars() << std::endl;
}
rs.close();
stmt.close();
conn.close();
}
catch( Throwable t ) {
std::cerr << "*** Caught Java exception through Throwable: " << t.toString().to_chars() << std::endl;
}
What an EJB Integration Might Look Like
When you are faced with the problem of calling EJBs from another language, you—like most people—will probably think web services. After all, all modern application servers come with a built-in web services stack that's just waiting to be used.
Why do we propose a different solution? Well, for one thing we like to sell our product, but a JunC++ion-based solution truly has many very attractive technical characteristics which we would like to point out to you. EJB and C++ can work very well with each other if you allow JunC++ion to be the middleman.
Architecture
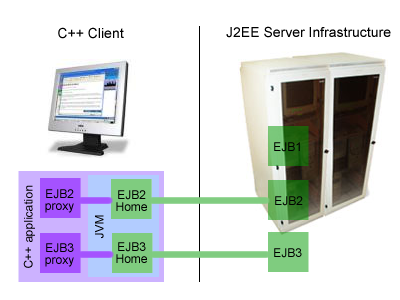
As the image on the right shows, you have your regular J2EE server infrastructure on the server side. You do not need to make any deployment or configuration changes to enable the C++ client use case from the server's perspective.
On the client side, you obviously have the C++ application that wishes to communicate with the J2EE server. Inside the application, you have generated proxy types for the EJB home interfaces and any other Java types that you wish to use. The C++ developer wrote code directly against these generated bindings, almost as if the J2EE server were really publishing a C++ based infrastructure rather than a Java infrastructure.
Under the hood, completely hidden from the C++ developer, the proxy types and the Codemesh runtime load a JVM into the C++ process and delegate (purple connectors) to the underlying Java objects, in this case the Java client-side bindings for the Enterprise Java Beans. These Java objects handle the communication with the server (green connectors). What's so remarkable about this picture?
- The developer is writing C++ code that happens to look a lot like Java code, but is otherwise blissfully unaware of the fact that there is any Java involved.
- The server is totally unaware that there is a C++ client. As far as it is concerned, it is communicating with a Java client, which happens to be "hosted" by a C++ application.
- Assuming you have Java clients for your server, you have to make absolutely no changes to your deployment. There are no security-related changes, no transactionalization-related changes, no firewall-related changes, no deployment-descriptor changes.
This kind of integration is just about as invisible and non-intrusive as possible.
JunC++ion users with this use case have found out through benchmarks on real applications and real application servers that this solution has much better performance than web services-based integration. Not only is there less overhead on the client side, but the server apparently scales better too when tested under load.
Development process
As part of your EJB deployment, your application server's deployment tool will typically offer to create a client deployment jarfile for you. You import this jar file (in a pinch you could also use the server-side jar) into the code generator and generate C++ proxy types for all Java types that you wish to use from within your C++ application. In the above picture, the developer decided not to generate proxies for EJB1, presumably because the C++ application does not need to use that particular EJB.
Once the C++ bindings have been generated (as source files), you can either build them into a library and use that library or you can add them directly to your C++ project, it's totally up to you. Regardless, you can now write C++ code such as this (it might look slightly different for your app server):
try { InitialContext ictx( _use_java_ctor ); Context myEnv = Context::dyna_cast( ictx.lookup( "java:comp/env" ) ); Object objref = myEnv.lookup( "ejb/SimpleConverter" ); ConverterHome home = ConverterHome::dyna_cast( PortableRemoteObject::narrow( objref, Class::forName( "com.myapi.ConverterHome" ) ) ); Converter currencyConverter = home.create(); BigDecimal param( "100.00" ); BigDecimal amount = currencyConverter.dollarToYen( param ); System::out.println( amount ); amount = currencyConverter.yenToEuro( param ); System::out.println( amount ); } catch( Exception & e ) { cerr << "Caught an unexpected exception!" ) << endl; e.printStackTrace(); }